Giant-scale information evaluation has develop into a transformative software for many industries, with purposes that embody fraud detection for the banking trade, medical analysis for healthcare, and predictive upkeep and high quality management for manufacturing. Nevertheless, processing such huge quantities of information could be a problem, even with the ability of recent computing {hardware}. Many instruments are actually obtainable to deal with the problem, with one of the vital in style being Apache Spark, an open supply analytics engine designed to hurry up the processing of very massive information units.
Spark offers a strong structure able to dealing with immense quantities of information. There are a number of Spark optimization methods that streamline processes and information dealing with, together with performing duties in reminiscence and storing incessantly accessed information in a cache, thus lowering latency throughout retrieval. Spark can also be designed for scalability; information processing could be distributed throughout a number of computer systems, rising the obtainable computing energy. Spark is related to many tasks: It helps quite a lot of programming languages (e.g., Java, Scala, R, and Python) and contains varied libraries (e.g., MLlib for machine studying, GraphX for working with graphs, and Spark Streaming for processing streaming information).
Whereas Spark’s default settings present an excellent place to begin, there are a number of changes that may improve its efficiency—thus permitting many companies to make use of it to its full potential. There are two areas to contemplate when interested by optimization methods in Spark: computation effectivity and optimizing the communication between nodes.
How Does Spark Work?
Earlier than discussing optimization methods intimately, it’s useful to take a look at how Spark handles information. The basic information construction in Spark is the resilient distributed information set, or RDD. Understanding how RDDs work is essential when contemplating easy methods to use Apache Spark. An RDD represents a fault-tolerant, distributed assortment of information able to being processed in parallel throughout a cluster of computer systems. RDDs are immutable; their contents can’t be modified as soon as they’re created.
Spark’s quick processing speeds are enabled by RDDs. Whereas many frameworks depend on exterior storage techniques resembling a Hadoop Distributed File System (HDFS) for reusing and sharing information between computations, RDDs assist in-memory computation. Performing processing and information sharing in reminiscence avoids the substantial overhead brought on by replication, serialization, and disk learn/write operations, to not point out community latency, when utilizing an exterior storage system. Spark is usually seen as a successor to MapReduce, the info processing element of Hadoop, an earlier framework from Apache. Whereas the 2 techniques share comparable performance, Spark’s in-memory processing permits it to run as much as 100 occasions quicker than MapReduce, which processes information on disk.
To work with the info in an RDD, Spark offers a wealthy set of transformations and actions. Transformations produce new RDDs from the info in current ones utilizing operations resembling filter()
, be part of()
, or map()
. filter()
creates a brand new RDD with components that fulfill a given situation, whereas be part of()
creates a brand new RDD by combining two current RDDs primarily based on a typical key. map()
is used to use a change to every aspect in a knowledge set, for instance, making use of a mathematical operation resembling calculating a proportion to each document in an RDD, outputting the leads to a brand new RDD. An motion, alternatively, doesn’t create a brand new RDD, however returns the results of a computation on the info set. Actions embody operations resembling depend()
, first()
, or gather()
. The depend()
motion returns the variety of components in an RDD, whereas first()
returns simply the primary aspect. gather()
merely retrieves the entire components in an RDD.
Transformations additional differ from actions in that they’re lazy. The execution of transformations shouldn’t be rapid. As a substitute, Spark retains observe of the transformations that must be utilized to the bottom RDD, and the precise computation is triggered solely when an motion is known as.
Understanding RDDs and the way they work can present worthwhile perception into Spark tuning and optimization; nevertheless, although an RDD is the muse of Spark’s performance, it may not be essentially the most environment friendly information construction for a lot of purposes.
Selecting the Proper Information Buildings
Whereas an RDD is the fundamental information construction of Spark, it’s a lower-level API that requires a extra verbose syntax and lacks the optimizations supplied by higher-level information buildings. Spark shifted towards a extra user-friendly and optimized API with the introduction of DataFrames—higher-level abstractions constructed on high of RDDs. The information in a DataFrame is organized into named columns, structuring it extra like the info in a relational database. DataFrame operations additionally profit from Catalyst, Spark SQL’s optimized execution engine, which may improve computational effectivity, probably bettering efficiency. Transformations and actions could be run on DataFrames the way in which they’re in RDDs.
Due to their higher-level API and optimizations, DataFrames are sometimes simpler to make use of and supply higher efficiency; nevertheless, on account of their lower-level nature, RDDs can nonetheless be helpful for outlining customized operations, in addition to debugging advanced information processing duties. RDDs supply extra granular management over partitioning and reminiscence utilization. When coping with uncooked, unstructured information, resembling textual content streams, binary information, or customized codecs, RDDs could be extra versatile, permitting for customized parsing and manipulation within the absence of a predefined construction.
Following Caching Greatest Practices
Caching is a vital method that may result in important enhancements in computational effectivity. Ceaselessly accessed information and intermediate computations could be cached, or continued, in a reminiscence location that permits for quicker retrieval. Spark offers built-in caching performance, which could be notably helpful for machine studying algorithms, graph processing, and every other software during which the identical information have to be accessed repeatedly. With out caching, Spark would recompute an RDD or DataFrame and all of its dependencies each time an motion was referred to as.
The next Python code block makes use of PySpark, Spark’s Python API, to cache a DataFrame named df
:
df.cache()
It is very important needless to say caching requires cautious planning, as a result of it makes use of the reminiscence sources of Spark’s employee nodes, which carry out such duties as executing computations and storing information. If the info set is considerably bigger than the obtainable reminiscence, otherwise you’re caching RDDs or DataFrames with out reusing them in subsequent steps, the potential overflow and different reminiscence administration points may introduce bottlenecks in efficiency.
Optimizing Spark’s Information Partitioning
Spark’s structure is constructed round partitioning, the division of enormous quantities of information into smaller, extra manageable models referred to as partitions. Partitioning allows Spark to course of massive quantities of information in parallel by distributing computation throughout a number of nodes, every dealing with a subset of the whole information.
Whereas Spark offers a default partitioning technique sometimes primarily based on the variety of obtainable CPU cores, it additionally offers choices for customized partitioning. Customers would possibly as a substitute specify a customized partitioning perform, resembling dividing information on a sure key.
Variety of Partitions
One of the vital vital components affecting the effectivity of parallel processing is the variety of partitions. If there aren’t sufficient partitions, the obtainable reminiscence and sources could also be underutilized. However, too many partitions can result in elevated efficiency overhead on account of job scheduling and coordination. The optimum variety of partitions is normally set as an element of the whole variety of cores obtainable within the cluster.
Partitions could be set utilizing repartition()
and coalesce()
. On this instance, the DataFrame is repartitioned into 200 partitions:
df = df.repartition(200) # repartition technique
df = df.coalesce(200) # coalesce technique
The repartition()
technique will increase or decreases the variety of partitions in an RDD or DataFrame and performs a full shuffle of the info throughout the cluster, which could be pricey when it comes to processing and community latency. The coalesce()
technique decreases the variety of partitions in an RDD or DataFrame and, in contrast to repartition()
, doesn’t carry out a full shuffle, as a substitute combining adjoining partitions to cut back the general quantity.
Dealing With Skewed Information
In some conditions, sure partitions might include considerably extra information than others, resulting in a situation generally known as skewed information. Skewed information may cause inefficiencies in parallel processing on account of an uneven workload distribution among the many employee nodes. To deal with skewed information in Spark, intelligent methods resembling splitting or salting can be utilized.
Splitting
In some circumstances, skewed partitions could be separated into a number of partitions. If a numerical vary causes the info to be skewed, the vary can typically be cut up up into smaller sub-ranges. For instance, if a lot of college students scored between 65% to 75% on an examination, the check scores could be divided into a number of sub-ranges, resembling 65% to 68%, 69% to 71%, and 72% to 75%.
If a particular key worth is inflicting the skew, the DataFrame could be divided primarily based on that key. Within the instance code beneath, a skew within the information is brought on by a lot of information which have an id
worth of “12345.” The filter()
transformation is used twice: as soon as to pick all information with an id
worth of “12345,” and as soon as to pick all information the place the id
worth shouldn’t be “12345.” The information are positioned into two new DataFrames: df_skew
, which comprises solely the rows which have an id
worth of “12345,” and df_non_skew
, which comprises the entire different rows. Information processing could be carried out on df_skew
and df_non_skew
individually, after which the ensuing information could be mixed:
from pyspark.sql.capabilities import rand
# Cut up the DataFrame into two DataFrames primarily based on the skewed key.
df_skew = df.filter(df['id'] == 12345) # comprises all rows the place id = 12345
df_non_skew = df.filter(df['id'] != 12345) # comprises all different rows
# Repartition the skewed DataFrame into extra partitions.
df_skew = df_skew.repartition(10)
# Now operations could be carried out on each DataFrames individually.
df_result_skew = df_skew.groupBy('id').depend() # simply an instance operation
df_result_non_skew = df_non_skew.groupBy('id').depend()
# Mix the outcomes of the operations collectively utilizing union().
df_result = df_result_skew.union(df_result_non_skew)
Salting
One other technique of distributing information extra evenly throughout partitions is so as to add a “salt” to the important thing or keys which can be inflicting the skew. The salt worth, sometimes a random quantity, is appended to the unique key, and the salted secret is used for partitioning. This forces a extra even distribution of information.
For instance this idea, let’s think about our information is cut up into partitions for 3 cities within the US state of Illinois: Chicago has many extra residents than the close by cities of Oak Park or Lengthy Grove, inflicting the info to be skewed.
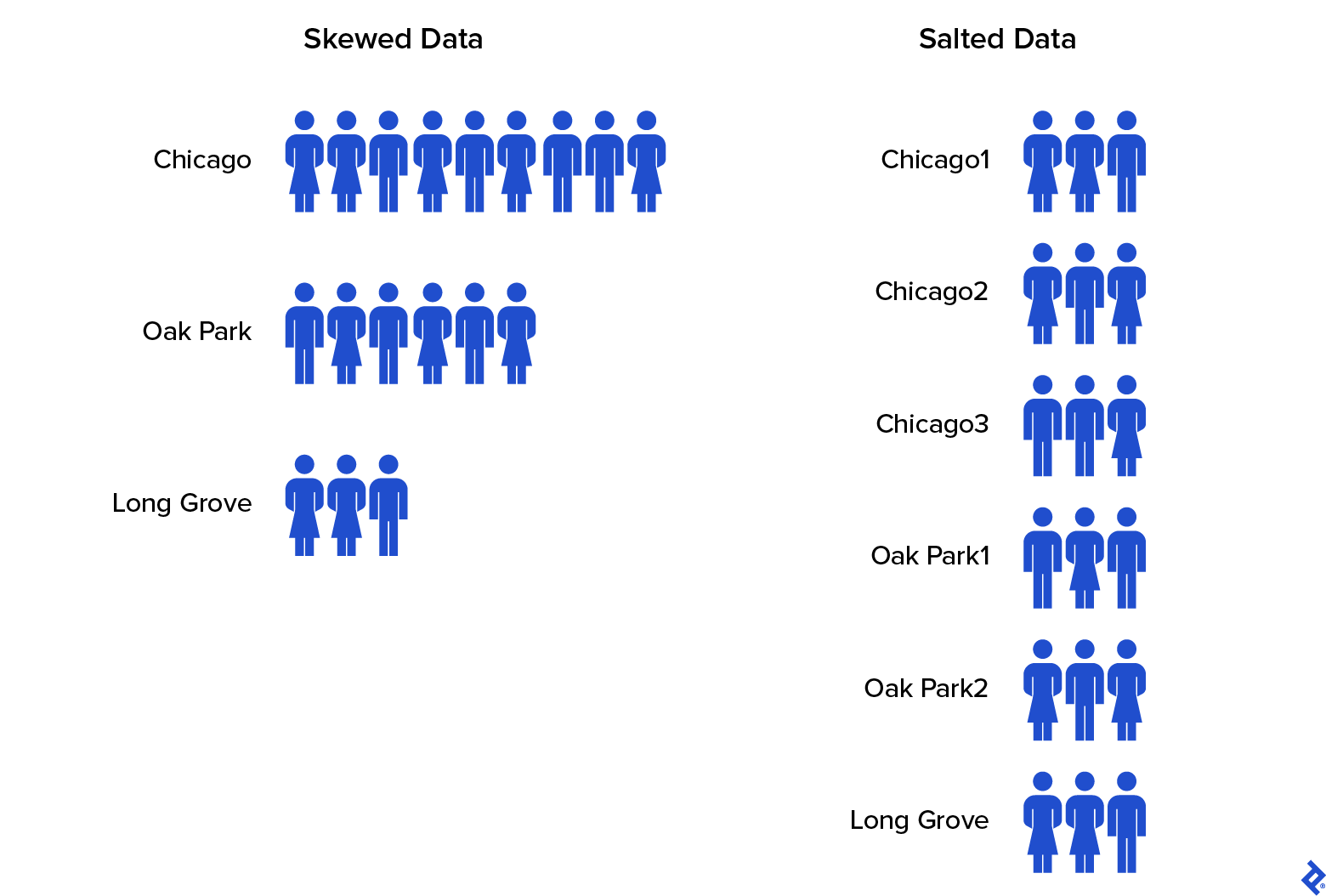
To distribute the info extra evenly, utilizing PySpark, we mix the column metropolis
with a randomly generated integer to create a brand new key, referred to as salted_city
. “Chicago” turns into “Chicago1,” “Chicago2,” and “Chicago3,” with the brand new keys every representing a smaller variety of information. The brand new keys can be utilized with actions or transformations resembling groupby()
or depend()
:
# On this instance, the DataFrame 'df' has a skewed column 'metropolis'.
skewed_column = 'metropolis'
# Create a brand new column 'salted_city'.
# 'salted_id' consists of the unique 'id' with a random integer between 0-10 added behind it
df = df.withColumn('salted_city', (df[skewed_column].solid("string") + (rand()*10).solid("int").solid("string")))
# Now operations could be carried out on 'salted_city' as a substitute of 'metropolis'.
# Let’s say we're doing a groupBy operation.
df_grouped = df.groupby('salted_city').depend()
# After the transformation, the salt could be eliminated.
df_grouped = df_grouped.withColumn('original_city', df_grouped['salted_city'].substr(0, len(df_grouped['salted_city'])-1))
Broadcasting
A be part of()
is a typical operation during which two information units are mixed primarily based on a number of widespread keys. Rows from two totally different information units could be merged right into a single information set by matching values within the specified columns. As a result of information shuffling throughout a number of nodes is required, a be part of()
could be a pricey operation when it comes to community latency.
In eventualities during which a small information set is being joined with a bigger information set, Spark affords an optimization method referred to as broadcasting. If one of many information units is sufficiently small to suit into the reminiscence of every employee node, it may be despatched to all nodes, lowering the necessity for pricey shuffle operations. The be part of()
operation merely occurs domestically on every node.
Within the following instance, the small DataFrame df2
is broadcast throughout the entire employee nodes, and the be part of()
operation with the massive DataFrame df1
is carried out domestically on every node:
from pyspark.sql.capabilities import broadcast
df1.be part of(broadcast(df2), 'id')
df2
have to be sufficiently small to suit into the reminiscence of every employee node; a DataFrame that’s too massive will trigger out-of-memory errors.
Filtering Unused Information
When working with high-dimensional information, minimizing computational overhead is crucial. Any rows or columns that aren’t completely required ought to be eliminated. Two key methods that scale back computational complexity and reminiscence utilization are early filtering and column pruning:
Early filtering: Filtering operations ought to be utilized as early as potential within the information processing pipeline. This cuts down on the variety of rows that must be processed in subsequent transformations, lowering the general computational load and reminiscence sources.
Column pruning: Many computations contain solely a subset of columns in a knowledge set. Columns that aren’t needed for information processing ought to be eliminated. Column pruning can considerably lower the quantity of information that must be processed and saved.
The next code reveals an instance of the choose()
operation used to prune columns. Solely the columns title
and age
are loaded into reminiscence. The code additionally demonstrates easy methods to use the filter()
operation to solely embody rows during which the worth of age
is larger than 21:
df = df.choose('title', 'age').filter(df['age'] > 21)
Minimizing Utilization of Python Consumer-defined Capabilities
Python user-defined capabilities (UDFs) are customized capabilities written in Python that may be utilized to RDDs or DataFrames. With UDFs, customers can outline their very own customized logic or computations; nevertheless, there are efficiency issues. Every time a Python UDF is invoked, information must be serialized after which deserialized between the Spark JVM and the Python interpreter, which results in further overhead on account of information serialization, course of switching, and information copying. This will considerably affect the pace of your information processing pipeline.
One of the vital efficient PySpark optimization methods is to make use of PySpark’s built-in capabilities every time potential. PySpark comes with a wealthy library of capabilities, all of that are optimized.
In circumstances during which advanced logic can’t be carried out with the built-in capabilities, utilizing vectorized UDFs, often known as Pandas UDFs, can assist to attain higher efficiency. Vectorized UDFs function on total columns or arrays of information, reasonably than on particular person rows. This batch processing typically results in improved efficiency over row-wise UDFs.
Take into account a job during which the entire components in a column have to be multiplied by two. Within the following instance, this operation is carried out utilizing a Python UDF:
from pyspark.sql.capabilities import udf
from pyspark.sql.varieties import IntegerType
def multiply_by_two(n):
return n * 2
multiply_by_two_udf = udf(multiply_by_two, IntegerType())
df = df.withColumn("col1_doubled", multiply_by_two_udf(df["col1"]))
The multiply_by_two()
perform is a Python UDF which takes an integer n
and multiplies it by two. This perform is registered as a UDF utilizing udf()
and utilized to the column col1
throughout the DataFrame df
.
The identical multiplication operation could be carried out in a extra environment friendly method utilizing PySpark’s built-in capabilities:
from pyspark.sql.capabilities import col
df = df.withColumn("col1_doubled", col("col1") * 2)
In circumstances during which the operation can’t be carried out utilizing built-in capabilities and a Python UDF is critical, a vectorized UDF can supply a extra environment friendly different:
from pyspark.sql.capabilities import pandas_udf
from pyspark.sql.varieties import IntegerType
@pandas_udf(IntegerType())
def multiply_by_two_pd(s: pd.Collection) -> pd.Collection:
return s * 2
df = df.withColumn("col1_doubled", multiply_by_two_pd(df["col1"]))
This technique applies the perform multiply_by_two_pd
to a complete sequence of information directly, lowering the serialization overhead. Notice that the enter and return of the multiply_by_two_pd
perform are each Pandas Collection. A Pandas Collection is a one-dimensional labeled array that can be utilized to signify the info in a single column in a DataFrame.
Optimizing Efficiency in Information Processing
As machine studying and large information develop into extra commonplace, engineers are adopting Apache Spark to deal with the huge quantities of information that these applied sciences must course of. Boosting the efficiency of Spark entails a spread of methods, all designed to optimize the utilization of accessible sources. Implementing the methods mentioned right here will assist Spark course of massive volumes of information far more effectively.